Variable & Identifiers
Now that you understand data and values, let's talk about identifiers and variables. We'll understand how variables are created in JavaScript and what an identifier is. We'll also learn the rules for creating variables and how to use them. Once this tutorial is complete, you'll be in a position to easily create variables.
Understanding how to properly name and use variables is fundamental in programming as they allow us to store and manipulate data, making our code more dynamic and flexible.
Identifiers in programming are names used to represent variables, functions, objects, or any other entity, adhering to specific naming rules within the language. Think of it as a label that helps you refer to and work with different pieces of your program. Below are the some common naming conventions for identifiers:
- Must start with a letter, an underscore (_), or a dollar sign ($)
- After that initial character, you can use as many letters, digits (numbers), underscores, or dollar signs as you want
- Variables and functions usually begin with lowercase letters.
- Constants should be in uppercase. For example: RATE
- Avoid using reserved words as identifier names.
- When naming variables and functions, use camelCase. For example: myAge, rateOfInterest.
- Constants, on the other hand, prefer snake_case. Use underscores (_) to separate words. For example: INTEREST_RATE
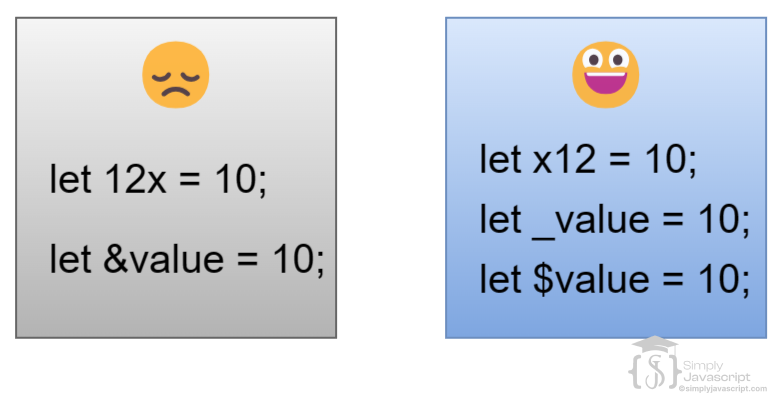
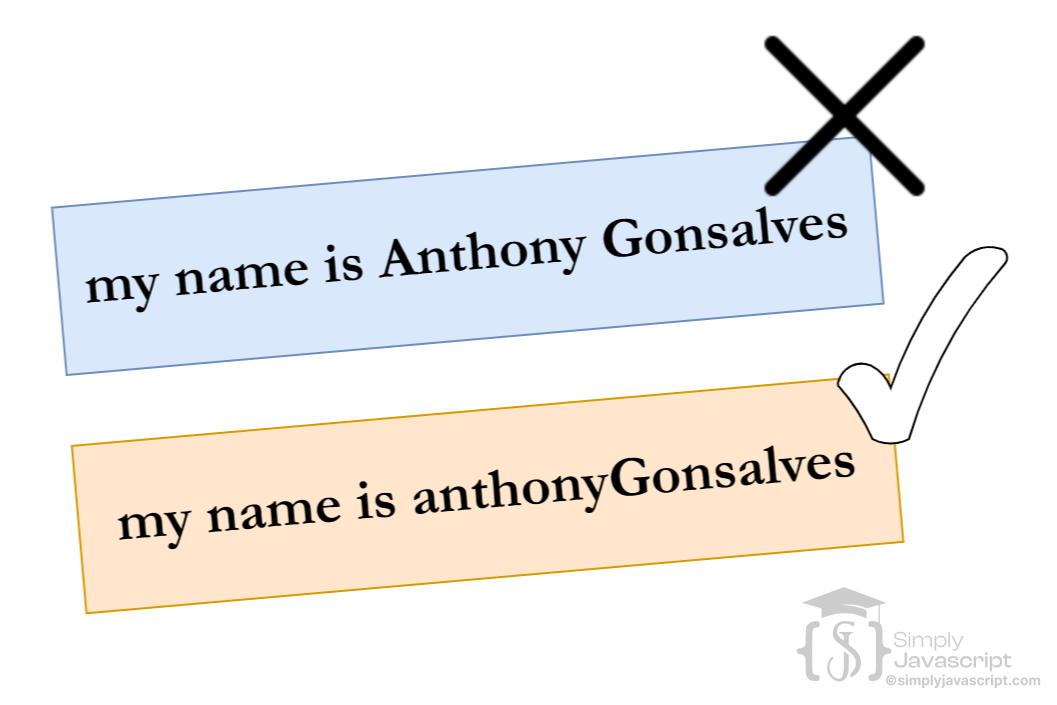
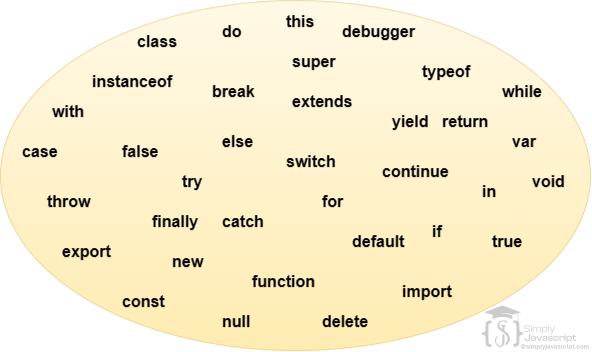
Reserved keywords in programming languages are predefined terms that hold special meanings or functionalities and cannot be used as identifiers for variables or other user-defined entities within the code. You can think of them as already claimed for certain jobs within JavaScript.
If you try to use these reserved keywords as names for your variables or functions, JavaScript will get confused—it’ll throw a syntax error, basically saying, "Hey, you can't use that word here—it's reserved for something else!" So, it's like a no-go zone for naming your stuff. For example: function, if, for etc.
A variable in programming is a named storage location that holds data, allowing its value to be referenced and manipulated throughout the program. It's super handy because you can store data in these containers and then use that data whenever you need it in your program.
To create a variable, you use special keywords like var or let, followed by a name of your choice, which you'll use to refer to the value stored in that container. For example, look at this code:
let age = 30; //30 is value and age is variable
Once you've created a variable like this, you can access its value and even change it as your program runs. That means you can give age a new value or even change the type of data it holds while your program is running.
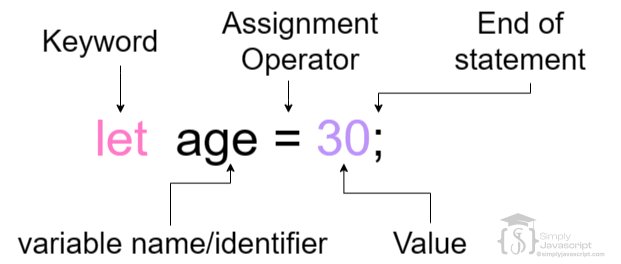
The var keyword in JavaScript is used to declare variables, with a broader scope than let and const, allowing redeclaration and can be accessed throughout the function or globally. For example:
var val = 300;
console.log(val); //prints 300
console.log(age); //allowed and value will be undefined here
var age;
var val = 400; //redeclaration allowed
let and const were introduced in later versions of JavaScript (ES6) to address certain issues that developers faced with var. var is still functional in JavaScript, but let and const offer clearer and more predictable behavior in scoping and preventing certain bugs. Developers often prefer using let or const over var due to the advantages like hoisting and scoping.
Below are the some important points for var:
- Can be redeclared
- Can be reassigned
- Accessible in global scope (attached to Window object)
- Delcared using camelCase. For example: myAge
- Has function scope
- Does not have block scope
- Hoisting occurs
- Temporal Dead Zone (TDZ) applies
The let keyword in JavaScript declares block-scoped variables that can be reassigned within their scope, offering more control than var over variable redeclaration and scoping. This helps to prevent accidental conflicts with other variables that may have the same name, and makes the code easier to read and understand. For example:
let x = 10;
let x = 20;
console.log(x);//SyntaxError: 'x' has already been declared
Below are the some important points for let:
- Cannot be redeclared
- Can be reassigned
- Accessible in global scope
- Delcared using camelCase. For example: myAge
- Has function scope
- Has block scope
- Hoisting occurs
- Temporal Dead Zone (TDZ) applies
A constant in programming is a named value that cannot be altered during the execution of the program, maintaining its assigned value throughout.
When you declare a constant variable using the const keyword in programming, you must assign a value to it at the time of declaration. Once assigned, this value cannot be changed throughout the program's execution. If you attempt to declare a constant without assigning a value to it immediately, you'll encounter an error. For example:
const PI = 3.14;
//below code will result in an error
PI = 3.14159;
const RATE; //this will result in an error as value is not assigned.
Below are the some important points for constant:
- Cannot be redeclared
- Constants should be in uppercase
- Cannot be reassigned
- Accessible in global scope
- Constants delcared using snake_case. Use underscores (_) to separate words. For example: INTEREST_RATE
- Has function scope
- Has block scope
- Hoisting occurs
- No Temporal Dead Zone (TDZ)
Description | var | let | const |
---|---|---|---|
Launch Year | Since beginning | 2015 (ES6) | 2015 (ES6) |
Accessible in Global Scope | Yes (Attached to Window Object) | Yes (Not attached to window object) | Yes (Not attached to window object) |
Function Scope | Yes | Yes | Yes |
Block Scope | No | Yes | Yes |
Redeclaration | Yes | No | No |
Reassigned | Yes | Yes | No |
TDZ | No | Yes | Yes |
Hoisting | Yes | Yes | Yes |
Identifiers in JavaScript:
- Names given to variables, functions, or other code parts. They must start with a letter, underscore (_), or dollar sign ($) and can continue with letters, numbers, underscores, or dollar signs.
Naming Conventions:
- Variables/functions start with lowercase; constants use uppercase.
- Avoid using reserved keywords as identifiers.
- Use camelCase for variables/functions and snake_case for constants.
Reserved Keywords:
- Pre-defined words in JavaScript with specific language meanings. They cannot be used as identifiers for user-defined parts of the code.
Variable:
- Containers holding data in JavaScript. Created using keywords like "var," "let," or "const" followed by a chosen name. Variables can change types/values during execution and can exist without an immediate value (defaulting to "undefined").
var:
- Declares variables with functional scope, allowing updates and re-declarations within the scope.
let:
- Declares variables with block scope, preventing conflicts and making code more readable.
Constants:
- Similar to variables but once initialized with "const," their value cannot change. Commonly used for values that shouldn't change throughout the program.
const:
- Declares constants with block scope, preventing value changes or re-declarations after initialization.
- What are identifiers in JavaScript? Can you provide examples of valid and invalid identifiers?
- Explain the rules and conventions for creating identifiers in JavaScript. What naming conventions should be followed while naming variables or functions?
- Explain the concept of reserved keywords.
- Define variables in JavaScript. How are they used to store and manipulate data within a program?
- What are constants in JavaScript? How do they differ from variables, and why might you use constants in your code?
- Explain the purpose and usage of the let keyword in JavaScript. How does let differ from var in terms of scope and hoisting?
- Explain the usage and behavior of the var keyword in JavaScript. What are the scope and hoisting implications of using var?
- Describe the role and behavior of the const keyword in JavaScript. What restrictions does it impose on variable reassignment, and how does it relate to immutability?