Features
Before proceeding further in this JavaScript series, it's essential to understand the features of JavaScript that set it apart from other high-level languages. Knowing these distinct features will provide us with a basic understanding of JavaScript.
Note: In this tutorial, we've covered essential JavaScript features. If these concepts seem challenging now, don't worry. As the course progresses, we'll provide detailed explanations to enhance your understanding. Feel free to revisit or skip this section for now.
High-level programming languages use human-friendly syntax and structures, simplifying coding by abstracting technical details, and making it easier to write programs compared to lower-level languages. High-level languages, like Python, Java, C#, and JavaScript, work in a similar way. They're designed to be super understandable for us, which makes coding a lot more efficient and enjoyable.
Garbage collection is an automatic memory management process in programming that frees up memory by identifying and removing unused or no longer referenced objects. and making your life as a coder a whole lot easier.
Imagine this: while you're coding in JavaScript, your program is using memory. Sometimes, it doesn't need all the memory it initially grabbed. That's where garbage collection swoops in! It keeps an eye on the memory your program isn't using anymore and clears it out. This way, you don't have to stress about manually allocating and deallocating memory, which can be a real headache and a cause of bugs and memory leaks.
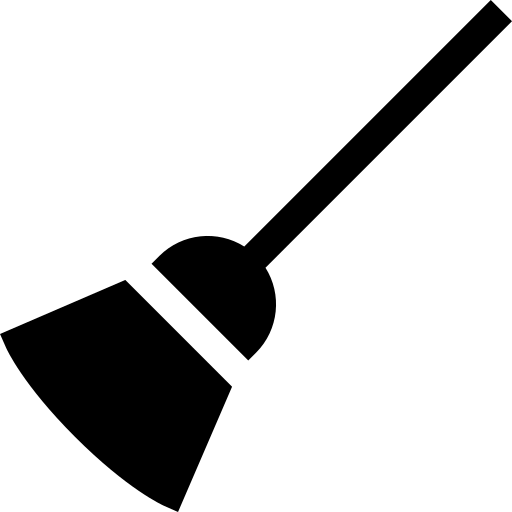
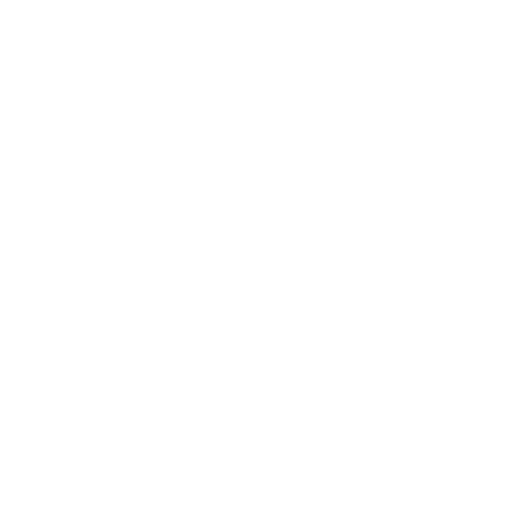
JavaScript, an interpreted language, utilizes a Just-In-Time compiler for optimizing code during runtime, enhancing performance by dynamically translating and executing code as needed. JavaScript is an interpreted language, which means it doesn't get translated into machine code before it runs. Instead, it's read and executed line by line by these super cool engines like V8 (in Chrome and Node.js), SpiderMonkey (in Firefox), or Chakra (in Edge).
Imagine this: you have your JavaScript code, and right when it's about to be used, BAM! It gets translated into machine code, the kind the CPU can understand lightning-fast. This dynamic translation at runtime is why it's called Just-In-Time. This makes JavaScript run large and complex applications way more efficiently.
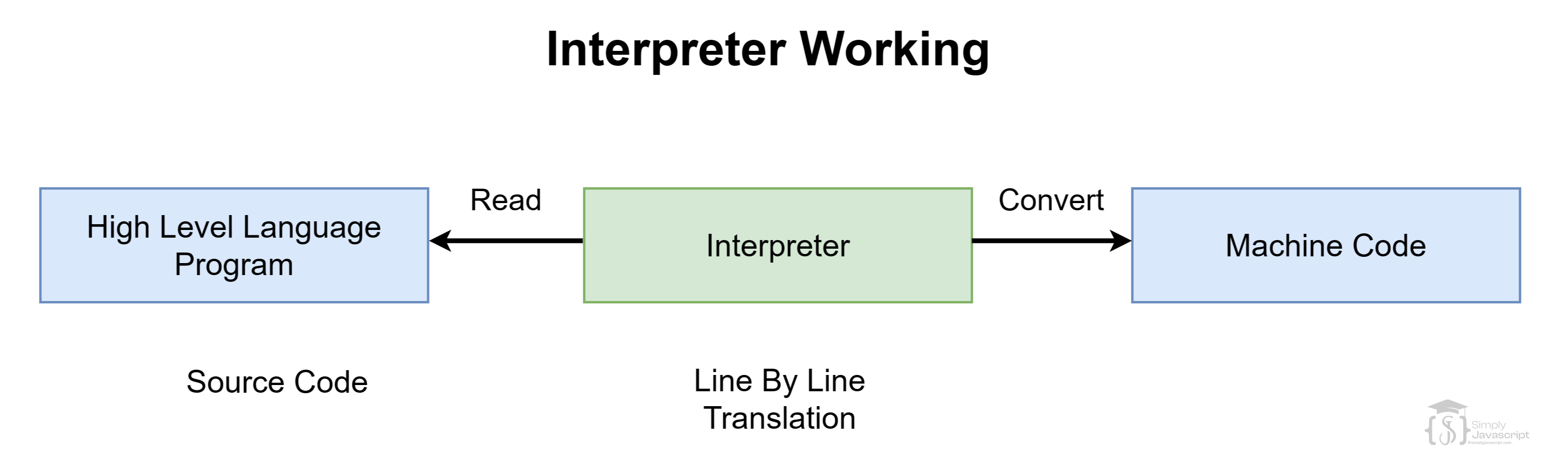
A multi-paradigm language accommodates different programming styles, allowing developers to use multiple approaches—such as event driven, object-oriented, and functional programming—in a single language. It supports several programming paradigms, which are different ways or styles of writing code.
You can use JavaScript to do things in different ways—whether you prefer the event driven approach of event driven programming, the organized object-oriented style, or even the functional way of handling things. It's like having multiple gears to shift into depending on what you need.
Prototype-based functions in JavaScript enable object creation where objects inherit properties and behaviors from prototypes, allowing dynamic modification and extension of object structures during runtime. You can think of a prototype as a model or template that new objects can follow. Every object in JavaScript has this hidden link to a prototype, which is accessed using __proto__.
When you're trying to grab a property or method from an object, JavaScript checks that object first. If it's not found there, it goes up the chain, checking the prototype, and keeps going until it either finds what it's looking for or reaches the top. This whole process is called "prototype-based inheritance."
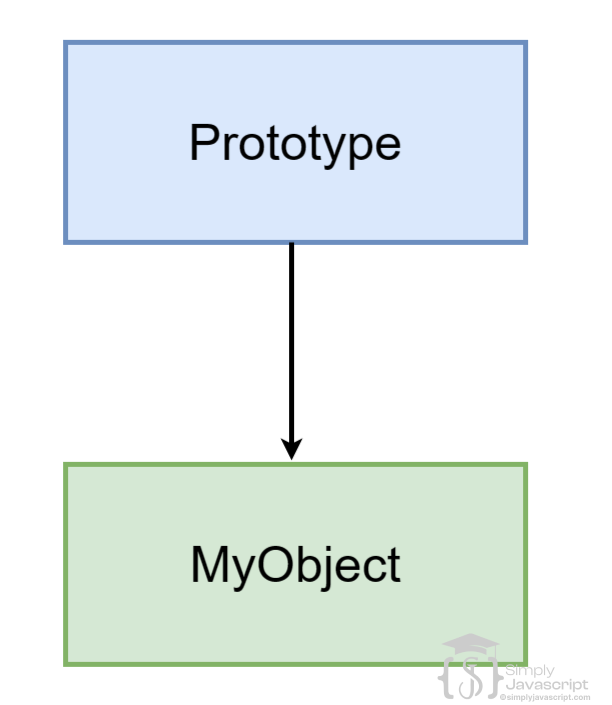
A first-class function is a function treated as a variable, enabling it to be passed around, returned, or assigned like any other value within the language. In JavaScript, a first-class function can do everything other values can do. Because of these first-class functions, things like higher-order functions and closures become possible. They're like the secret tools that help you write more expressive, flexible, and reusable code.
// Define a function
function greet(name) {
return "Hello, " + name + "!";
}
// Assign the function to a variable
var myFunction = greet;
// Call the function using the variable
var result = myFunction("John");
// Output the result
console.log(result);
In JavaScript, being dynamically typed means that variables can hold different types of data, and their types can change during runtime, allowing flexibility but potentially leading to unexpected behaviors or errors. In JavaScript, the type of a variable isn't fixed when you declare it. Instead, it's figured out while the program is running. That's what we call dynamic typing.
Imagine this: in some other languages, when you declare a variable as an integer, it'll always be an integer, no ifs, ands, or buts. But in JavaScript? That variable can hold anything—from numbers to strings or even change its type while the program is running. For instance, it could start as a number and later turn into a string without causing a trouble.
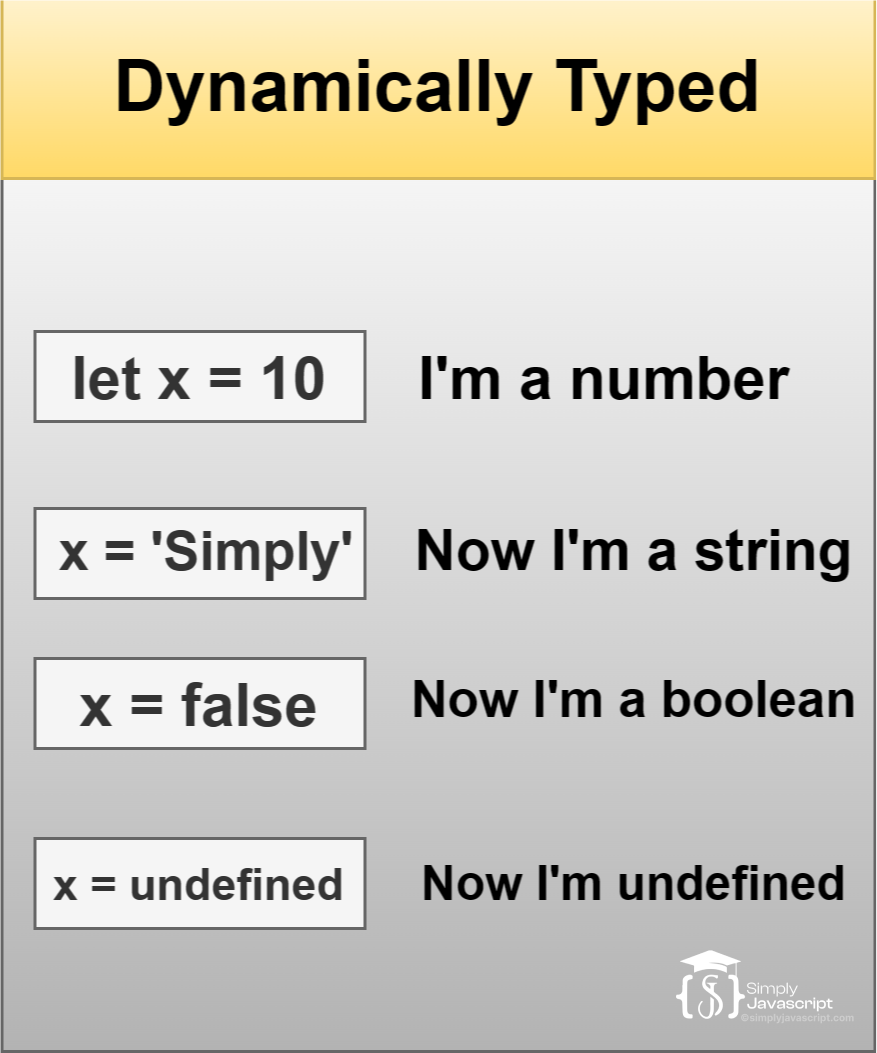
let x = 10; // type will be number
x = "SimplyJavascript"; //type will be string
x = false; //type will be boolean
JavaScript's single-threaded nature executes one set of instructions at a time, ensuring synchronous operations, simplifying programming, but potentially causing blocking behavior in some cases. JavaScript operates on a single-threaded model, which means it handles one task at a time. It's like having a single lane on a road—only one car moves ahead at any given moment. This setup in JavaScript contrasts with multi-threaded languages, where multiple tasks can happen all at once, like a highway with many lanes where cars zoom past simultaneously.
But when JavaScript encounters long or heavy tasks, it can cause performance hiccups. Picture a traffic jam where one slow car holds up the rest. To tackle this, JavaScript offers solutions like Web Workers and Asynchronous programming. These tools allow heavy tasks to happen in the background, away from the main thread, so they don't block other important operations.
JavaScript's non-blocking event loop manages tasks asynchronously, enabling efficient handling of I/O operations without stalling the main thread, enhancing performance in web applications. It is the mechanism that keeps things moving smoothly without blocking the whole show.
Imagine it as a vigilant loop constantly checking a message queue for any new tasks or events to handle. When a long-running async task comes along, JavaScript doesn't put everything on hold. Instead, it cleverly puts that task into the message queue and carries on with other things—like responding to user clicks or handling various events. When that task finally finishes, its result joins the queue, and the event loop takes it up in the next cycle.
This way, your program stays super responsive and doesn't freeze up waiting for those time-consuming tasks to finish. This technique is called "asynchronous programming". It's like being able to handle multiple tasks at once without dropping any balls. This is a big reason why JavaScript is great for creating web apps that feel quick and perform smoothly.
JavaScript can work on all sorts of devices and systems without needing changes. It's because it lives in web browsers, which are like the language interpreters of the internet. They're made to work on different devices and systems. So, when JavaScript runs in a browser, it doesn't care about what device or system it's on. It's like talking to any device or system without needing any special changes.
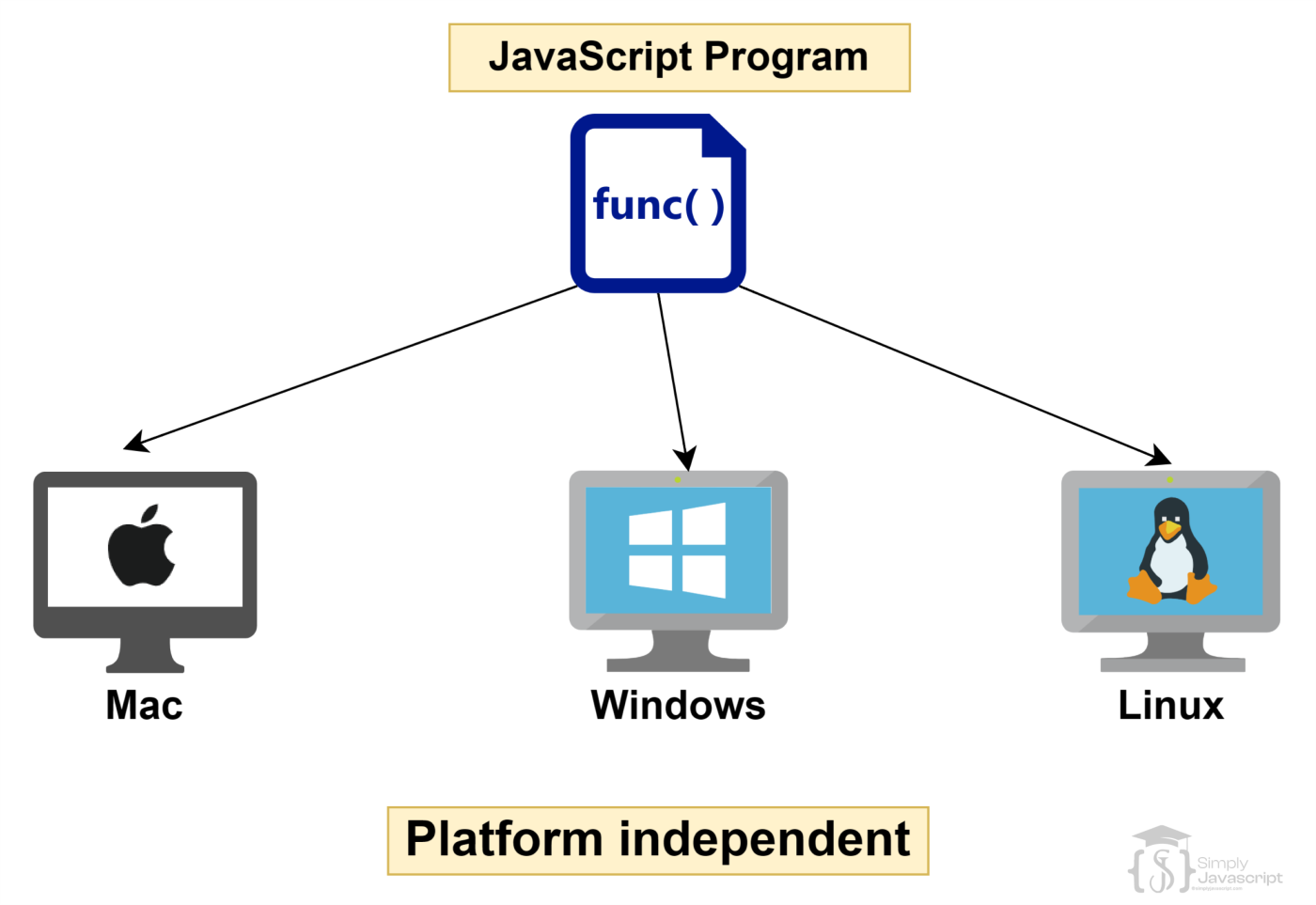
High-Level Language:
- Human-friendly, easier to read and write compared to low-level languages.
- Allows for efficient problem-solving without delving into hardware specifics.
- Prominent high-level languages include Python, Java, C#, and JavaScript.
Garbage Collection:
- Manages memory by clearing unused memory segments automatically.
- Reduces manual memory management concerns, preventing bugs and memory leaks.
- Commonly employed in languages like Java, C#, Python, and Ruby.
Interpreted Language with JIT Compilation:
- JavaScript is interpreted line by line but employs Just-In-Time (JIT) compilation for speed.
- Converts JavaScript code into machine code just before execution, enhancing performance.
- Implemented by engines like V8, SpiderMonkey, and Chakra in browsers.
Multi-Paradigm Language:
- Supports multiple coding styles like object-oriented, functional, and event-driven programming.
- Provides flexibility for developers to choose the best approach for a particular task.
- Offers support for object-oriented features like classes, objects, and inheritance.
Prototype-Based Function and First-Class Functions:
- Utilizes prototypes as models for creating objects, enabling unique OOP concepts.
- Employs first-class functions treated as versatile values, enhancing flexibility.
Dynamically Typed/Dynamic:
- Variables' types are determined during runtime, allowing for variable type changes.
- Facilitates coding flexibility but might make early error detection challenging.
Single-Threaded:
- Executes one task at a time, simplifying code understanding but posing performance challenges for heavy tasks.
- Offers solutions like Web Workers and Asynchronous programming to manage heavy operations efficiently.
Non-Blocking Event Loop:
- Orchestrates asynchronous tasks, ensuring responsiveness without freezing the program.
- Utilizes a message queue to handle long-running async tasks, keeping the program smooth and responsive.
Platform Independence:
- Operates uniformly across different devices and operating systems within web browsers.
- Ensures consistent code execution without requiring specific adjustments for each platform.
- Explain all the JavaScript features in detail?
- Explain the concept of automatic memory management through garbage collection. How does it benefit developers in languages like JavaScript?
- Differentiate between interpreted and compiled languages.
- How does JavaScript support multiple programming paradigms?
- Explain the concept of prototype-based inheritance in JavaScript. How does it differ from classical inheritance in languages like Java or C++?
- Define first-class functions in programming languages. How does JavaScript treat functions as first-class citizens, and what advantages does it offer?
- How does JavaScript's dynamic typing differ from statically typed languages?
- Explain what it means for JavaScript to be single-threaded. What are the implications of this for concurrency and performance in web applications?
- What is the event loop, and how does it help with asynchronous programming?
- Explain the significance of JavaScript being platform-independent.
- What is Just-In-Time compilation ? Explain benefits.