Setup
Before diving deeper, let's get your JavaScript workspace set up for effective learning. This step is essential to ensure a smooth journey into understanding and implementing JavaScript's powerful capabilities. Let's begin by configuring your environment for optimal learning!
A web browser is a software application used to access and display content on the internet, interpreting and rendering web pages. To run JavaScript, all you need is a web browser.
If you have a laptop or desktop, chances are you already have a browser installed. If not, you can easily install a modern browser like Chrome, Mozilla Firefox, etc. These browsers will assist you in your web development journey and learning JavaScript.
To install browsers, you can use a search engine like Google by typing "Download and install" followed by the browser name. Download Google Chrome
An IDE (Integrated Development Environment) is a software package that combines essential tools for writing and managing code in one interface. Think of it as a one-stop shop where you can write your code, debug it for errors, test it out, and manage the whole project.
IDE typically includes a code editor that helps you write your code more easily by providing features like autocomplete, syntax highlighting (which makes different parts of the code stand out), and sometimes even suggestions for fixing errors. Additionally, it often has built-in tools for running and testing your code without needing to switch to another program.
Visual Studio Code (VSCode) is a feature-rich, open-source code editor widely used for web development. While coding is possible in basic text editors like Notepad, they lack advanced features found in dedicated Integrated Development Environments (IDEs) such as Sublime Text.
For this course, we'll utilize Visual Studio Code due to its extensive features, open-source nature, and free accessibility. You can download VSCode by visiting the following link and proceeding with the installation. Download VSCode
After download, for a straightforward setup, create a folder and open it in VSCode by navigating to the File menu, then selecting "Open Folder...". Choose the folder where you intend to write your code. Begin writing and creating files within this folder to facilitate future development.
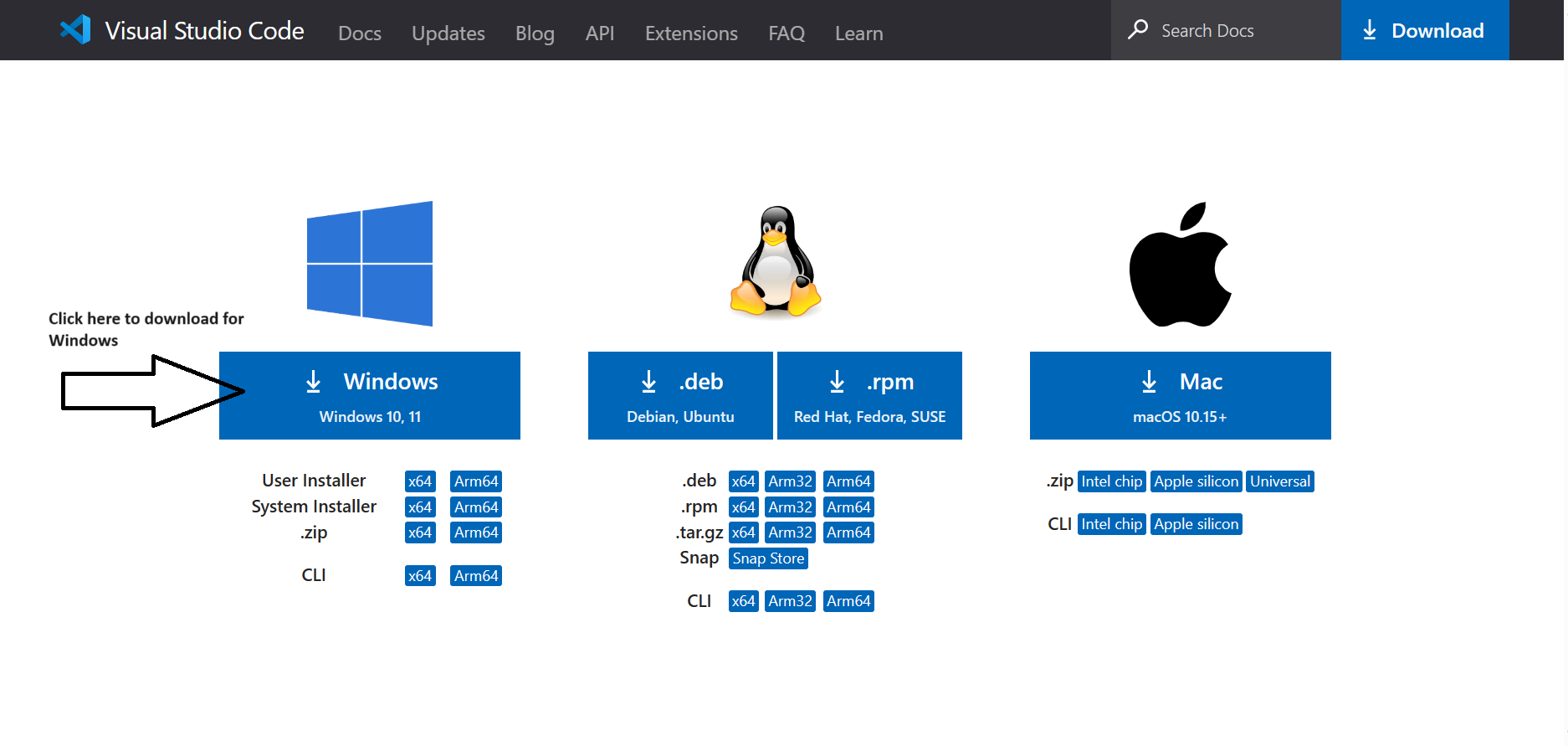
For more details about Visual Studio Code click here or watch our explanatory video.
An extension in VSCode is like adding a new feature to your program. It's like getting extra tools or abilities to customize and improve your coding experience. Just like apps on a phone, they give more options and make coding easier. You can pick and add extensions to customize your coding experience.
For this web development course, you can download the extensions below, which should be sufficient for you. However, you can download more extensions as per your needs.
-
Live Server: The Live Server
extension in VSCode creates a live preview of your
website. It sets up a local server and updates your
changes instantly in the browser, making it easy to
see how your code affects the website without manual
refreshes.
-
Prettier: Prettier is like an
automatic formatter for your code. It helps make
your code look clean and consistent by automatically
adjusting the formatting—things like
indentation, spacing, and line breaks. It
supports multiple programming languages and can be
integrated into various code editors, making your
code more readable and maintaining a consistent
style across your project.
The separation of concerns principle in JavaScript refers to the practice of separating different parts of a program or application into distinct and independent units, with each unit responsible for a specific functionality or concern. This allows for better organization, maintainability, and scalability of the codebase.
For example, in a Web application, the concerns of layout, styling, and scripting can be separated into different modules or files. This allows for easier testing, debugging, and updating of individual parts of the application without affecting the rest of the codebase.
- Step 1: Open VSCode
- Step 2: Create or Open a folder
- Step 3: Create HTML File - Create a new file. Save it with a .html extension (extension tell the operating system and software programs what type of file it is and how to handle it.), for example, index.html. To follow the seperation of concerns prinicple, we will not write JavaScript code directly in the HTML file but will instead link to a separate JavaScript file.
- Step 4: Create JavaScript File - Create a new file and save it with a .js extension, such as script.js. This will contain your JavaScript code. Now open script.js and start writing some JavaScript code. For example:
- Step 5: Run in a Browser/Start Live Server - Open index.html in a web browser. You can right-click on the index.html file and choose "Open with" to select your preferred browser. Check the browser's console (usually accessible by right-clicking, selecting "Inspect," and navigating to the "Console" tab) to see if the JavaScript code is running (Hello, SimplyJavaScript! should appear in the console).
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>My JavaScript Page</title>
</head>
<body>
//Your HTML content goes here
<script src="script.js"></script>
</body>
</html>
console.log("Hello, SimplyJavaScript!");
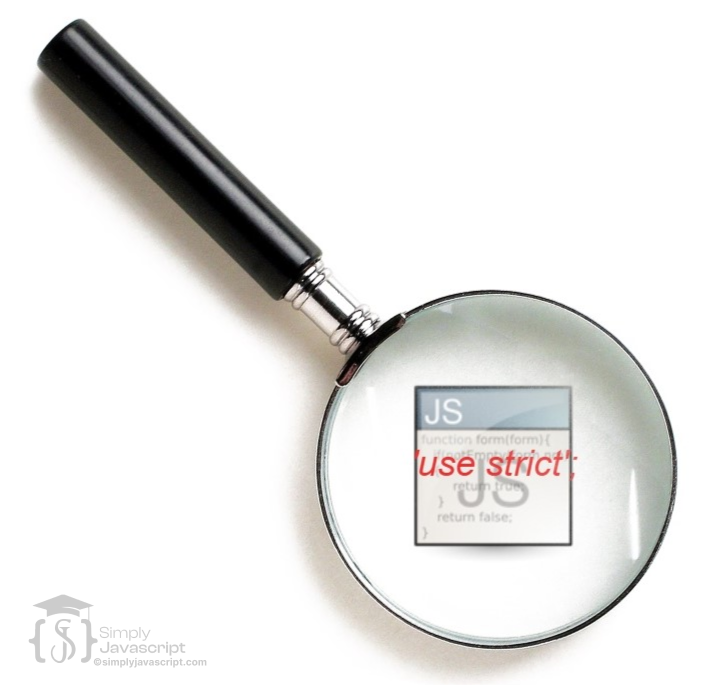
"use strict" is a way to make JavaScript behave in a stricter, more secure way. It helps catch coding mistakes and makes JavaScript work more predictably. For example: without "use strict," forgetting to declare a variable can create a global variable unintentionally but with "use strict," it throws an error for undeclared variables. For example:
// This code works without 'use strict'
x = 10; // It creates a global variable 'x' accidentally.
console.log(x); // Output: 10
'use strict';
x = 10; // This will throw an error because 'x' isn't declared.
console.log(x); // This line won't be executed due to the error above.
You can place the "use strict"; directive at the beginning of a script or at the beginning of a function. For example:
//At the beginning of a script:
"use strict";
// Your JavaScript code goes here
//At the beginning of a function:
function myFunction() {
"use strict";
// Code for this specific function
}
Placing it at the beginning of a script enables strict mode for the entire script, while putting it within a function makes strict mode specific to that function's scope.
Note: There are many other advantages of using 'use strict' which we will cover in a separate blog.A developer tool in a web browser is a set of features and utilities designed to assist web developers in building, debugging, and optimizing websites and web applications. These tools are often included as part of the browser itself and can be accessed through the browser's menu or by using keyboard shortcuts. To open them, just press F12 or Ctrl+Shift+I (Cmd+Option+I on Mac) in most browsers.
You can inspect and change how elements look and behave. They also catch mistakes in your code, acting like a detective to find and fix errors. Developer tools show you what's happening behind the scenes and where things might be slowing down. Overall, they're a lifesaver for developers, making the job of building, fixing, and optimizing websites much easier!
Note: There are many useful tools in developer tools that help us in web development, which we will cover in a separate blog and discuss all the tools in detail.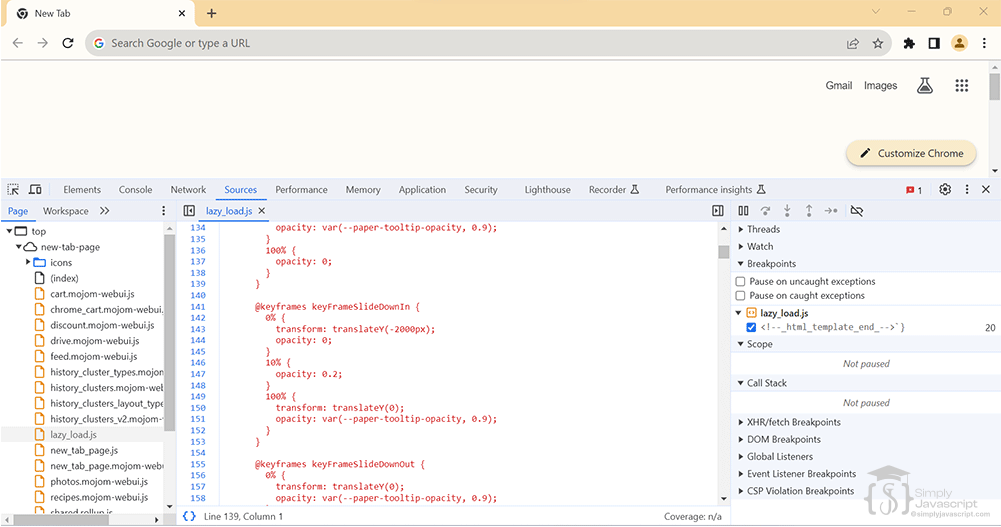
Web Browser:
- A software application to access and display internet content, interpreting and rendering web pages. Install modern browsers like Chrome or Firefox for web development and JavaScript learning.
IDE (Integrated Development Environment):
- Software combining tools for coding and project management. Acts as a one-stop platform for writing, debugging, and running code. Visual Studio Code (VSCode) is recommended for its extensive features and accessibility.
Visual Studio Code:
- A feature-rich, open-source code editor widely favored for web development, offering advanced features beyond basic text editors like Notepad.
Extensions:
- They are like adding apps to your phone, they customize and enhance your coding experience, offering extra tools and abilities for web development.
Separation of Concerns Principle:
- Divides systems into distinct parts for managing different aspects independently. This enhances organization and maintainability in software design, aiding in easier testing and updating of specific functionalities.
use strict:
- "use strict" in JavaScript enforces stricter rules, enhancing security by catching coding mistakes and ensuring more predictable behavior, such as preventing unintentional creation of global variables.
Developer Tools:
- Significance of developer tools as built-in toolkits for web developers.
- Functions including inspection, code debugging, performance analysis, and optimization.
- What is a web browser?
- What is a web page?
- What is web?
- What is an IDE?
- What is seperation of concerns principle?
- What are the difference place where we can write JavaScript code?
- What is the significance of "use strict"?
- Describe the importance of developer tools in web development. Name a few commonly used developer tools and their primary functions.
- Why don't we use "use strict" explicitly in frameworks like Angular and React?