DataTypes
In any programming language, data is one of the most crucial elements. You could say that without data, the computer world would be of no use. Data can include various types of information, such as your bank account details, personal information, and much more. The process of handling data in programming involves understanding of different data types, such as numbers, strings, arrays, and objects.
We use variables to hold and manipulate data, and these variables have specific types to accommodate different kinds of information. For instance, a variable holding a person's age might be a number type, while a variable storing their name would likely be a string type.
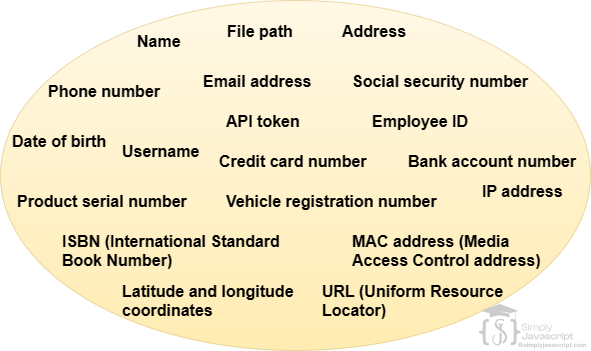
In programming, "data" refers to information or values used in computations, operations, or storage within a system or program. Data in JavaScript comes in various types like numbers, strings (which are just texts), booleans (true/false), arrays (lists of stuff), objects (bundles of related data), and other types to represent different kinds of information.
Each type of data plays a role. Numbers do math, strings handle texts, booleans make decisions, arrays group things together, and objects organize data in a structured way.
In programming, "value" refers to a specific piece of data, representing a singular or composite element stored or manipulated within a system. There are a few types of values in JavaScript:
- Primitive values: like numbers, strings, booleans etc.
- Complex values: Objects and arrays fall into this category.
- Special values: These include values, which can be used to represent missing or unknown values
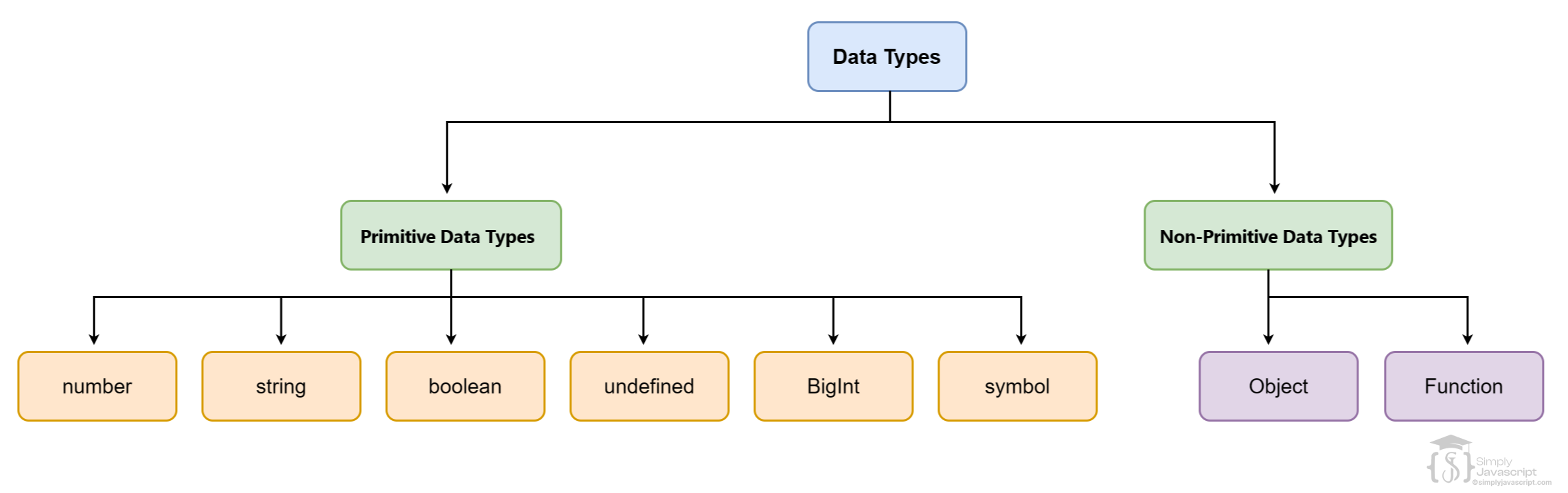
A data type in programming defines the kind of values a variable (type of a container) can hold, specifying its characteristics, storage requirements, and the operations that can be performed on it.
In JavaScript, data types are like the categories that classify the various flavors of data, dictating how they behave and what they can do. In JavaScript, we can check the type of any data using the typeof operator. Below are the two main categories of data types:
- Primitive Data Types: These are the fundamental building blocks. You've got numbers, strings, booleans, undefined, BigInt for handling big integers, and symbols for creating unique identifiers. Each of these holds a single value and has its own unique properties.
- Non-Primitive values: These are a bit more complex—they're like versatile containers. Functions and objects fall into this category. Functions are blocks of code you can reuse, and objects are these super flexible structures that hold key-value pairs.
console.log(typeof 1); // 'number'
console.log(typeof 'hello'); // 'string'
console.log(typeof true); // 'boolean'
console.log(typeof {}); // 'object'
console.log(typeof []); // 'object'
console.log(typeof function() {}); // 'function'
console.log(typeof null); // 'object'
console.log(typeof undefined); // 'undefined'
console.log(typeof NaN); // 'number'
Primitive data types in programming are basic data types not derived from other types, representing simple values like numbers, strings, booleans, or symbols. They include:
- Number: Handles numeric values, whether they're whole numbers (integers) or numbers with decimals (floating-point values). Example: 10, -92, 10.50
- String: Represents sequences of characters, whether it's a word, a sentence, or even just a single letter. Strings are enclosed in single or double quotes. Example: "SimplyJavaScript", 'John'.
- Boolean: Holds either true or false. It's the language's decision maker, determining what's right and what's wrong. Example: true, false.
- Symbol: Represents unique and unchangeable values. It's like a secret code that no one can replicate. Example: Symbol("A")
- undefined: Shows up when a value hasn't been assigned yet. It's like an empty slot, waiting to be filled. Example: undefined
- BigInt: Handles really, really big integers beyond what regular numbers can manage. Example: BigInt(9454354354354354355)
Non-primitive data types in programming are complex data structures that can hold multiple values and are not directly accessed, including objects, functions, and more. They include:
- Object: Represents a collection of key-value pairs. Objects are enclosed in curly braces and can hold a mix of different data types.
- Function: Represents a block of code that can be executed/called/invoked.
// Object
let obj = {
myName: "learnjavascript",
age: 21
};
// function
function sum() {
console.log(1+2);
}
Truthy and falsy values in JavaScript describe values that evaluate to either true or false in conditional statements. Truthy values are the values that the language treats as "true" when it's in a situation where it needs a true or false answer—like in a condition or a boolean operation. Pretty much every value in JavaScript is considered truthy except below six types of values :
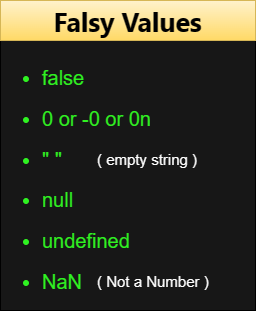
If you've got a value that's not on this list, JavaScript will treat it as truthy. It's a handy way for JavaScript to evaluate things when it's checking conditions or doing logical operations. Remembering these falsy values can be pretty helpful when you're writing code that involves conditions or boolean logic! For example:
if (NaN) {
console.log("Yes");
} else {
console.log("No"); //Answer : because NaN is a falsy value
}
if (10) {
console.log("Yes"); //Answer : because 10 is a truthy value
} else {
console.log("No");
}
Coercion in programming refers to the automatic conversion of one data type to another, often done by the language to facilitate operations or comparisons between different types. It happens when you're working with different types of data and using certain operators or actions.
For example, let's say you're dealing with numbers and strings. Sometimes, if you add a number to a string or try to compare them using certain operators, JavaScript will do this automatic conversion for you. It's like when you add a number to a string. JavaScript steps in and converts that number to a string so it can join it with the other string. Or when you're comparing a boolean to a number, JavaScript might convert that boolean to a number to make the comparison work.
This type of automatic conversion is what we call type coercion. It can be super useful, but it's also something to be aware of because it might not always give you the results you expect! Understanding how JavaScript handles type coercion helps you write more predictable and reliable code.
//Examples
//The Number 10 is converted to string '10' and then '+' concatenates both strings
var x = 10 + "20";
var y = "20" + 10;
//The Boolean value true is converted to string 'true' and then '+'concatenates both the strings
var z = true + "10";
console.log(x, y, z); //1020 2010 true10
//string to number conversion
var w = 10 - "5";
var x = 10 * "5";
var y = 10 / "5";
var z = 10 % "5";
console.log(w, x, y, z); //5 50 2 0
//The Boolean value true is converted to number 1 and then operation is performed
var x = true + 2;
//The Boolean value false is converted to number 0 and then operation is performed
var y = false + 2;
console.log(x, y); // 3 2
When you're not sure about how JavaScript might implicitly convert data types, you can take control by using constructors like Number(), String(), or Boolean(). Let's say you've got a value and you want to make absolutely sure it's a number, string, or boolean—no surprises! You can use these constructors to explicitly convert that value into the data type you want.
Number() converts to a number, String() to a string, and Boolean() to a boolean. It's a way to take charge and be clear about the type of data you're working with, especially when you're uncertain about how JavaScript might handle type conversions implicitly. Using these constructors gives you more control and helps you avoid any unexpected behavior in your code.
//number to boolean
let ans = Boolean(10);
console.log(ans); //true
ans = Boolean(0);
console.log(ans); //false
//number to string
ans = String(10);
console.log(ans); //'10'
//string to number
let x = "20";
console.log(Number(x) + 20);
console.log(+x); //another way to convert string to number
ans = Number("learnjavascript"); //Wrong Conversion
console.log(ans); //Not a Number (NaN)
//string to boolean
ans = Boolean('Simply');
console.log(ans); //true
ans = Boolean('');
console.log(ans); //false
Data in JavaScript:
- Represents various types such as numbers, strings, booleans, arrays, objects, etc. Each type serves a specific purpose, functioning like tools in a toolkit, aiding in different tasks within a program.
Values:
- Categorize data in JavaScript. Primitive types include numbers, strings, booleans, undefined, BigInt, and symbols. Non-primitive types encompass objects and functions. JavaScript's dynamic typing allows variable types to change during execution.
Variable:
- A container storing information. Created using keywords like var, let, or const, they can hold different values or change types during program execution.
Truthy and Falsy Values:
- JavaScript treats certain values as either true or false in conditions. Falsy values include false, 0, "", null, undefined, and NaN. All other values are considered truthy.
Type Coercion:
- JavaScript's automatic conversion between different data types, often occurring during operations involving different types. Understanding coercion helps write predictable code.
Manual Type Conversion:
- Constructors like Number(), String(), and Boolean() allow explicit conversion between types, offering control over data type handling, especially when unsure about implicit conversions.
- Define 'data' and 'value' in programming. How are they related to each other?
- What is Data type?
- What role do data types play in handling values within a program?
- What are the different types of data types in JavaScript?
- What are the differences between Primitive and Non-primitive data types ?
- Explain the concept of truthy and falsy values in JavaScript. Can you provide examples of values that are considered truthy and falsy?
- Explain type coercion in JavaScript. How does implicit coercion differ from explicit coercion?
- Explain manual type conversion.