Before advancing in the JavaScript series, it's crucial to understand how we can observe the execution of a program, like seeing the result of an action we've taken. In JavaScript, we can achieve this using methods (A method is a piece of code that is designed to perform a specific task) like console.log(), alert(), write() innerHTML(), and more. Let's delve into each of these methods one by one and see how they work when used.
console.log() outputs messages or data to the console, aiding in debugging or displaying information during JavaScript execution. This function is incredibly helpful for developers because it lets you check your code as it runs. You just use console.log() and pass in whatever you want to see—whether it's a simple string like "Hello World" or even variables and expressions.
For instance, if you want to check what's stored in a variable or see if a particular part of your code is running as expected, just write it into console.log() and watch the magic happen. It'll show up in the browser's developer console, giving you a sneak peek at what's going on behind the scenes.

//Printing in same line
console.log("learnjavascript", "simplyjavascript", 2+2);
//Printing in new line
console.log("learnjavascript");
console.log("simplyjavascript");
document.write() outputs content directly into an HTML document, enabling immediate rendering of text or HTML onto a webpage. This is a handy method during development because it lets you toss in some text or HTML right onto your page.
But it's a bit risky to use, especially after the page has fully loaded it might wipe out everything on the page and replace it with what you're trying to add. Imagine erasing a whiteboard before writing something new—it's a bit like that!
That's why most folks recommend steering clear of document.write() in production code. Instead, for adding content after the page has loaded, the DOM API is the way to go! You've got cool methods like document.createElement() and document.appendChild() that let you create new elements and add them to your web page without the risk of accidentally erasing things.
document.write("Hello World");
document.write("Hello SimplyJavaScript</br>Hello Learners"); //br will print Hello Learners in the new line
window.alert() displays a pop-up dialog box in the browser window, showcasing a message to the user, commonly used for notifications or prompts in JavaScript. When you call window.alert() and pass in a message, it creates this little pop-up window with the message you've provided. It's a simple "OK" button affair—once the user clicks "OK," they're back to the web page.
But here's the thing: while alerts are handy for grabbing attention, they can be a bit disruptive. They pause the JavaScript execution until the user interacts with the alert, which might not be the smoothest experience, especially for more complex web applications.
That's why, for less intrusive and more user-friendly notifications, developers often prefer alternatives like the browser's notification API or even custom notification components. These methods provide a more seamless experience for users without interrupting the flow of the application.
window.alert("Hello");
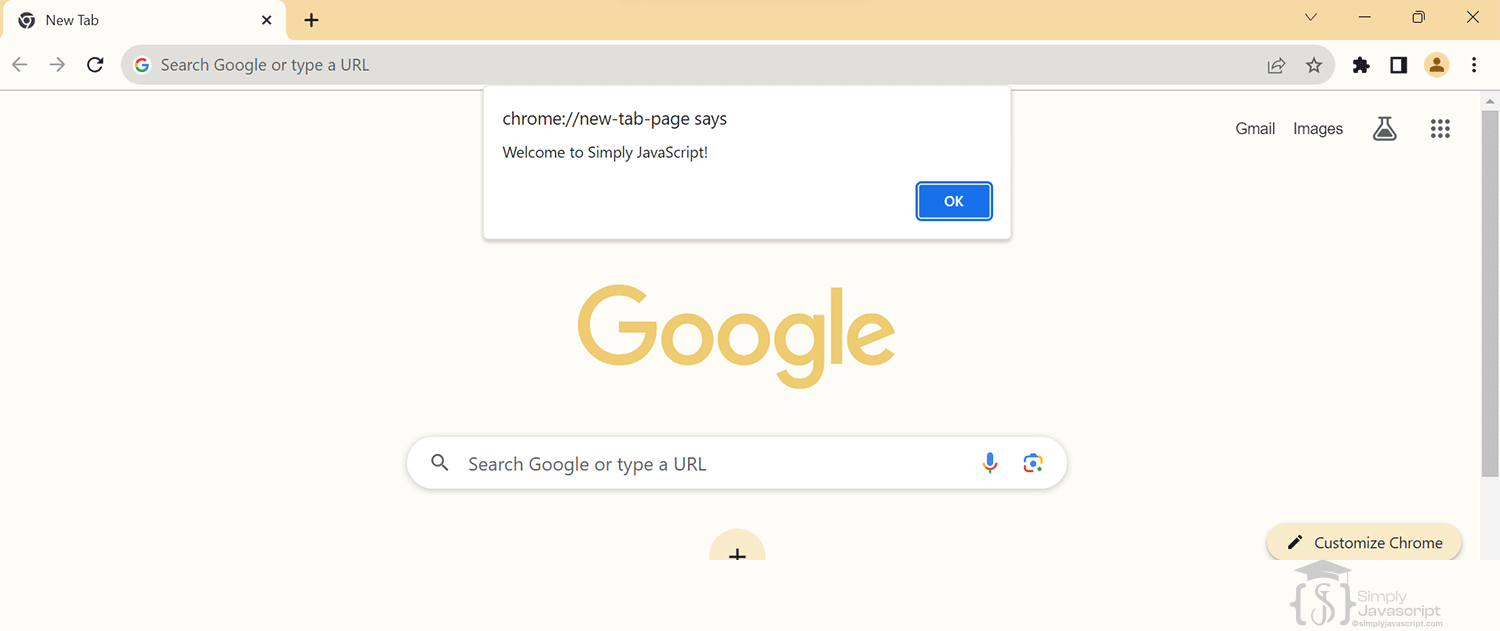
innerHTML is a property used in JavaScript to access or modify the HTML content within an element on a webpage, allowing dynamic changes to the displayed content. When you access an element's innerHTML, you're basically getting or setting the HTML content that lives within that element. Imagine it as the gateway to the guts of an element—you can see what's already there or swap it out with something new.
For instance, if you want to check what's currently nestled inside an element, you just access its innerHTML. Likewise, if you want to update that content—whether it's text, images, or anything in HTML—you can simply modify the innerHTML property and the element will show the updated content. Just remember, while innerHTML is super handy for making quick changes, it's also important to handle it with care to ensure your changes don't accidentally mess up the structure or functionality of the element!
Note: We'll delve into innerHTML in detail when we cover the topic of the Document Object Model (DOM). Right now, you just need a basic understanding that innerHTML is one way to display output in JavaScript.
<div id='test'></div>
document.getElementById('test').innerHTML = '<span>Hello World</span>';
Topic | console.log() | document.write() | window.alert() | innerHTML |
---|---|---|---|---|
Function | Used for logging messages | Writes directly to document | Displays a popup dialog | Manipulates HTML content |
Output | Outputs to the console | Outputs directly to the HTML | Displays as a popup alert | Dynamically updates HTML |
Usage | Commonly for debugging | Used for quick HTML output | Alerts user with information | Widely used for dynamic content rendering in the DOM |
Impact | Doesn't modify HTML content | Overwrites existing content | Interrupts user interaction | Allows dynamic content insertion safely |
console.log():
- A debugging tool in JavaScript that provides real-time insights into code execution. It allows developers to check variables, strings, and expressions, aiding in debugging and testing.
document.write():
- Quickly adds text or HTML to a web page. Useful during development but risky post-page load, potentially replacing existing content. For production, DOM methods like createElement() and appendChild() are safer for adding content.
window.alert():
- Displays messages in a dialog box, pausing JavaScript execution until user interaction. Useful for important messages but disruptive for complex applications. Alternatives like the browser's notification API offer less intrusive notifications.
innerHTML:
- Provides access to an HTML element's content. Allows retrieval or modification of content, making it a powerful tool for quick changes. However, caution is necessary to avoid unintentional structural or functional disruptions.
- What is the primary purpose of console.log() in JavaScript? How does it differ from other methods like console.error() or console.warn()?
- Can you describe situations where console.log() is particularly useful in debugging JavaScript code?
- Explain document.write() and potential drawbacks or limitations when using this method?
- When might you choose to use document.write() over other DOM manipulation methods like innerHTML or appendChild()?
- Explain window.alert(). How does it interact with user interaction and why might it be used sparingly?
- What are the advantages and disadvantages of using window.alert() for displaying messages compared to other methods like modals or custom UI elements?
- Explain the purpose and functionality of the innerHTML property in JavaScript. What are some considerations or best practices when using it to manipulate the DOM?
- How does innerHTML differ from other DOM manipulation methods, such as createElement() and appendChild()? When would you prefer one over the other?