Introduction
JavaScript is a high-level programming language primarily used for web development to create interactive and dynamic content on web pages. It can update content, animate images, control multimedia, and handle user interactions, all in real-time.
JavaScript can also run on servers, thanks to environments like Node.js, allowing developers to build and manage back-end functionality with the same language used on the front-end.
In JavaScript, a script refers to a piece of JavaScript code that executes tasks within a web page. These scripts can be embedded directly into HTML, run in response to events (like clicks or key presses), or manage data sent and received from a server.
Learning JavaScript can be an exciting journey, and while you can start with little to no programming experience, having a grasp on certain concepts and skills can significantly smooth your path. Here's a structured approach to getting ready for JavaScript:
Note: Before learning JavaScript, there are some terms that we should know. Let's understand them before diving into JavaScript.
A programming language is a set of rules and syntax used to create instructions that a computer can understand and execute to perform specific tasks or operations.
Programming languages serve as the communication tool between humans and computers and allow us to create various types of software and accomplish incredible tasks. Now, there are different types of these languages, but we can split them into two main categories:
- High-Level Programming Languages
- Low-Level Programming Languages
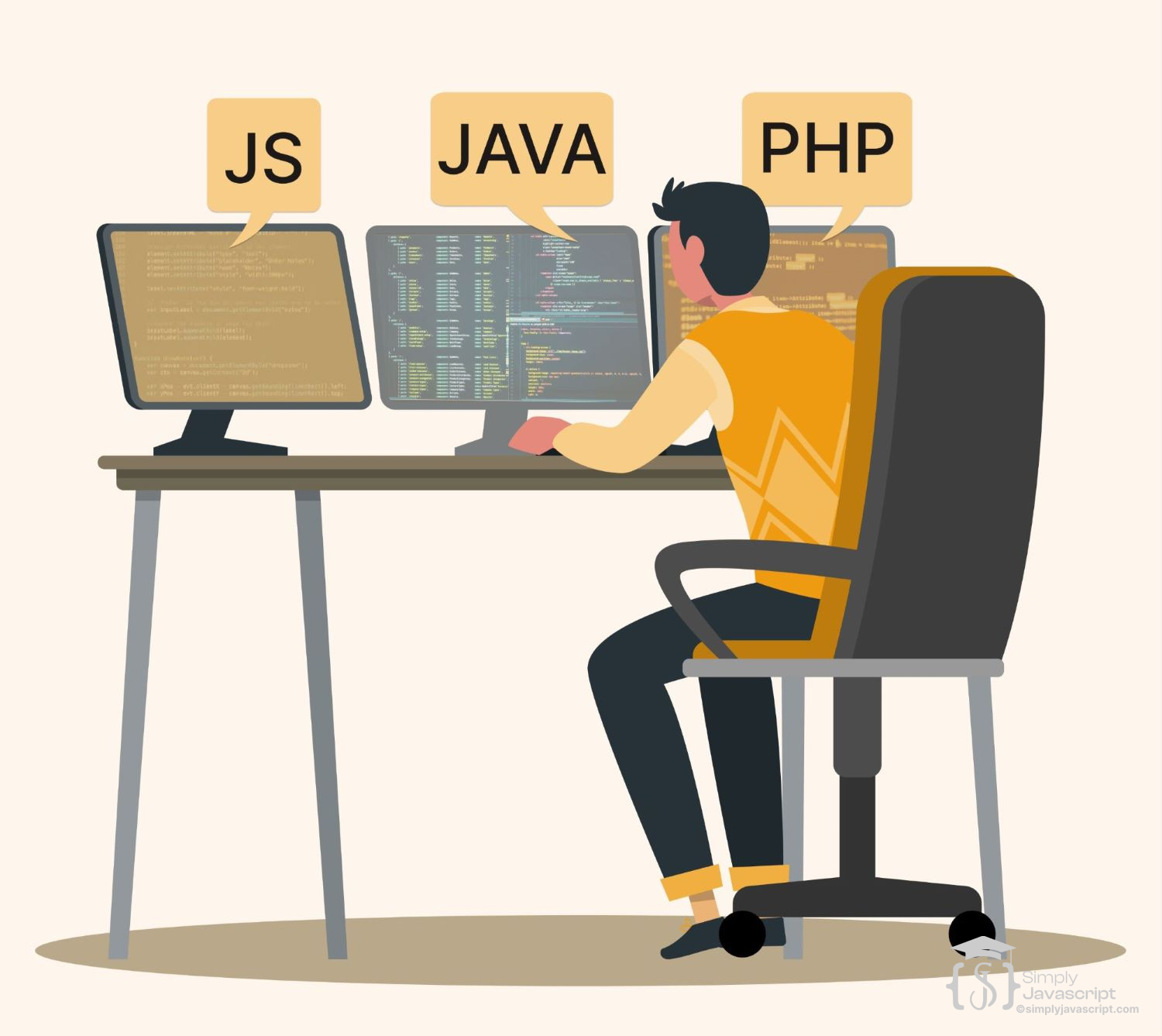
High-level programming languages use human-friendly syntax and instructions (english words), simplifying coding by abstracting technical details, and making it easier to write programs compared to lower-level languages. Computers don't speak our language and understand something called machine code.
These high-level languages need to be translated into that machine code before the computer can do its thing. That's where interpreters or compilers come in - they do the translation job. Python, Java, C++, and JavaScript are some examples of these high-level languages.
//Adding two numbers
let a = 20; // Assign 20 to a
let b = 40; // Assign 40 to b
let c = a + b; // Assign a + b to c
Low-level programming languages interact more directly with a computer's hardware, providing minimal abstraction and requiring a deeper understanding of hardware specifics for coding. Unlike high-level languages, which are easier for us humans to understand, low-level languages are a bit more trickier to work with and uses mnemonics instead of words.
There are a couple of types of low-level languages. One is assembly language, which is a bit easier for humans to deal with than pure machine code. Working in low-level languages requires a deep understanding of how computers are wired inside.
//Adding two numbers
moveq #20,d3 ;Move (quick) 20 to data reg 3
moveq #40,d4 ;Move (quick) 40 to data reg 4
move.l d3,d5 ;Move (long) reg 3 to reg 5
add.l d4,d5 ;Add (long) reg 4 to reg 5
Topic | High-level Language | Low-level Language |
---|---|---|
Syntax | Readable syntax with natural language-like keywords. | Cryptic mnemonics and direct representation of machine instructions. |
Abstraction | High level of abstraction. | Minimal abstraction, requiring understanding of hardware architecture. |
Portability | Excellent portability across platforms. | Platform-dependent, less portable due to hardware specificity. |
Development | Rapid development with built-in functions, reducing time. | Very complicated development, manual memory management, time-consuming. |
Debugging | Easier debugging | Challenging debugging, direct hardware interaction, limited error reporting. |
Frontend in web development is like the face of a website (collection of related web pages) - it's all about what users see and experience. It's the design, layout, and how everything interacts when someone visits a site. HTML, CSS, and JavaScript are the tools we use to create that fantastic user interface.
Note: In this JavaScript tutorial series, we'll focus on frontend applications. Backend applications will be covered in separate Node.js tutorials.
<!DOCTYPE html>
<html>
<head>
<title>Simply JavaScript</title>
</head>
<body>
<h1>Welcome To Simply JavaScript!</h1>
</body>
</html>
The backend of a website or application refers to the server-side operations and functionality responsible for managing and processing data, handling requests from the frontend, and interacting with databases or external services.
Databases and server-side programming languages like Java, Python, and Ruby—these are the tools for building this backend magic. Plus, there are frameworks like Express.js, Ruby on Rails, and Django that help us work faster and smarter.
Backend
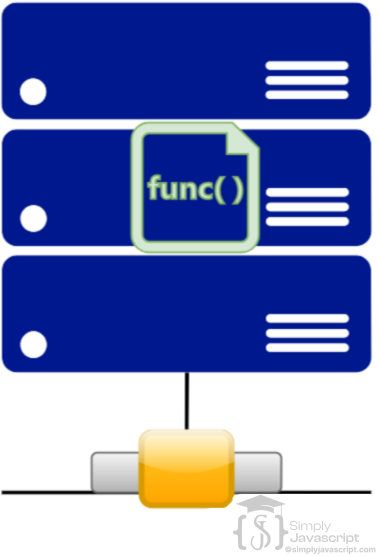
//Hello SimplyJavaScript program in Java
class Hello {
public static void main(String[] args) {
System.out.println("Hello SimplyJavaScript!");
}
}
Topic | Frontend | Backend |
---|---|---|
User Interface | Deals with user interaction and visual presentation. | Manages server-side operations and data processing. |
Technologies | Utilizes HTML, CSS, and JavaScript for client-side rendering. | Relies on server-side languages like Python, Java, or Node.js for server logic. |
Responsiveness | Focuses on ensuring a seamless and intuitive user experience across devices. | Ensures efficient data handling, authentication, and server communication. |
Interaction | Concerned with user input, form validation, and client-side interactions. | Handles database queries, server requests, and application logic execution. |
Performance | Affects the speed and responsiveness of the user interface. | Impacts the efficiency and scalability of the application's backend operations. |
Visibility | Interacts directly with users, visible within the browser. | Operates behind the scenes, often invisible to end-users. |
HTML (Hypertext Markup Language) is the standard language used to create web pages. It structures content on the internet by defining the meaning and layout of elements using tags and attributes.
Whenever you open a website in a web browser, the browser reads and interprets the HTML code, then shows the web page to the user based on how it's structured in that code. A website is made up of several web pages. For instance, this introduction.html is also a web page created using HTML, CSS, and JavaScript. When someone opens an HTML document in a web browser,
CSS (Cascading Style Sheets) is a styling language used to control the presentation and layout of HTML documents, allowing for the customization of colors, fonts, spacing, and overall visual appearance of web pages.
CSS separates the page's look (handled by CSS) from its structure (handled by HTML). This separation makes it super easy to update the design without messing with the actual content. Plus, with CSS, we can make designs that adapt to different screens, ensuring they look great on any device, and we can also make things more accessible for everyone.
JavaScript is a programming language used for creating interactive elements on web pages, enabling dynamic content and user interactions.
With JavaScript, we can make websites and web apps super interactive and dynamic. Think of it as the magic ingredient that adds behaviors to web pages. It responds to what users do—clicking, typing, creates cool animations, changes how stuff looks, and even manipulates what's on the page.
It's like a team effort! HTML sets up the skeleton of a webpage, CSS comes in to give it the stylish looks, and then JavaScript steps up to make things lively and interactive. They're the dream team of web development, working together to create sites that look great and do awesome stuff!
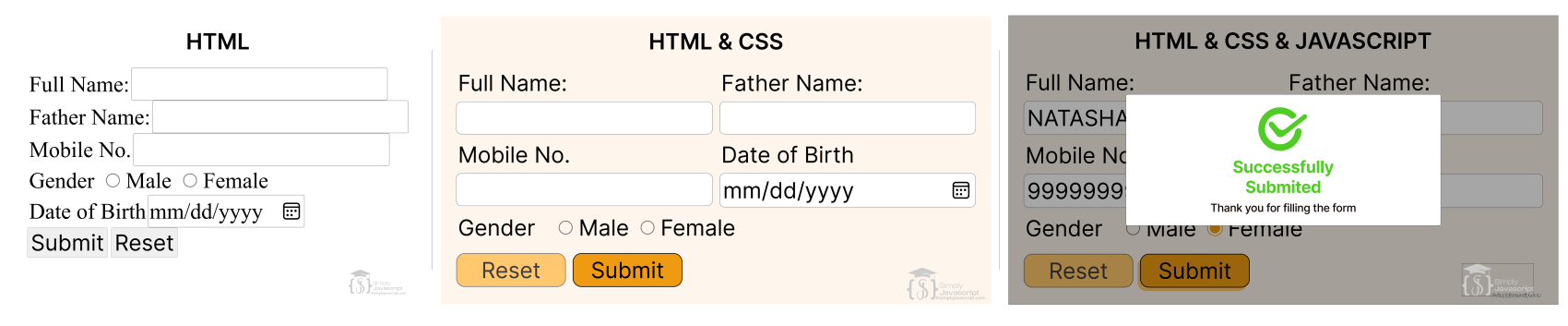
Javascript was designed to keep things secure by not letting it dive too deep into the technical details like memory or computer processing stuff. Why? Because it was made to play nice with web browsers, which don't need that kind of access.
While Javascript can do all sorts of fancy tricks like reading and writing files, talking to other computers on the internet, and more using Node.js but when it's in your web browser, JavaScript becomes the ultimate web wizard! It can make web pages dynamic, interactive, and even chat with the web server behind the scenes.
For example, JavaScript within web browsers has the capability to:
- Make web pages interactive and dynamic.
- Change content and style on web pages.
- Respond to user actions like clicks and inputs.
- Fetch data from servers asynchronously.
- Create animations and validate form inputs.
- Control browser behavior and build web applications.
Contrarily, JavaScript within web browsers lacks the capability to:
- Access local files or manipulate servers directly.
- Interact with hardware devices.
- Perform multithreading.
- Access cross-domain resources without special permissions.
Introduction to JavaScript:
- JavaScript as the interactive wizard behind websites, bringing them to life.
- HTML as structure, CSS as style, and JavaScript as the dynamic force enabling interactions.
Programming Languages:
- Programming language: Instructions for computers.
- High-level languages: Human-readable instructions for computers.
- Low-level languages: Machine-readable instructions for computers.
High-Level Programming Languages:
- User-friendly languages further away from hardware details.
- Translation to machine code required for computer understanding.
- Examples: Python, Java, C++, JavaScript.
Low-Level Programming Languages:
- Direct interaction with computer hardware in its native language.
- Dealing with binary code closely understood by CPUs.
- Types: Assembly language, machine code.
Frontend Development:
- Concerns with the user-facing aspects of websites.
- Usage of HTML, CSS, and JavaScript for designing the user interface.
Backend Development:
- Backend as the engine under the hood, handling logic and data operations.
- Usage of databases and server-side programming languages.
HTML (Hypertext Markup Language):
- HTML as the structure builder of web pages.
- Use of HTML tags (e.g., p, h1) to format content and create structured web documents.
CSS (Cascading Style Sheets):
- CSS for enhancing the look and feel of web pages.
- Separation of page's look (CSS) from its structure (HTML).
- Ability to create responsive and accessible designs.
JavaScript:
- JavaScript's role in making web pages interactive and dynamic.
- Adding behaviors, animations, and manipulations to web content.
- Frontend and server-side capabilities; compatibility across browsers.
Role of HTML, CSS, and JS:
- Collaboration of HTML for structure, CSS for style, and JavaScript for interactivity.
Capabilities and Limitations of JavaScript:
- JavaScript, designed for web browsers, keeps things secure by avoiding deep technicalities.
- With Node.js, it becomes a versatile tool for web wizardry, enabling dynamic web experiences and server communication.
- What is a programming language?
- What is front-end?
- What is back-end?
- What is HTML?
- What is CSS?
- What is JavaScript?
- Differentiate between JavaScript and Java?
- Tell us about role of HTML, CSS, and JavaScript in Web development?
- What distinguishes a high-level programming language from a low-level programming language?
- Explain where low-level programming languages are preferred despite their complexity compared to high-level languages.
- Differentiate between frontend and backend development.
- What are the capabilities and limitations of JavaScript?