Popup Boxes
When you're making a website and you want to show messages or ask users for something, JavaScript has these cool methods: alert(), confirm(), and prompt(). They pop up boxes on the screen. The alert() shows a message, confirm() asks for confirmation, and prompt() asks for input. These methods make your website more fun and easy for users to use. So, when you need to talk to your website visitors, these tools are like your helpful buddies!
The alert box in JavaScript is displayed using window.alert(), providing a pop-up dialog displaying a message to the user. When you use alert(), you're basically telling the browser to show a warning message to the user. It pops up a little dialog box with a message you specify (but you can skip it if you want) and an "OK" button. It's like saying, "Hey, pay attention to this!"
alert("Welcome to Simply JavaScript!");
When this code runs, a little box will appear on the user's screen with your message, and they’ll need to click "OK" to make it go away. It's often used for quick warnings, prompts, or alerts to users. Just keep in mind, using it excessively can be a bit annoying, so use it wisely! It's like a tap on the shoulder, letting your users know something important is happening.
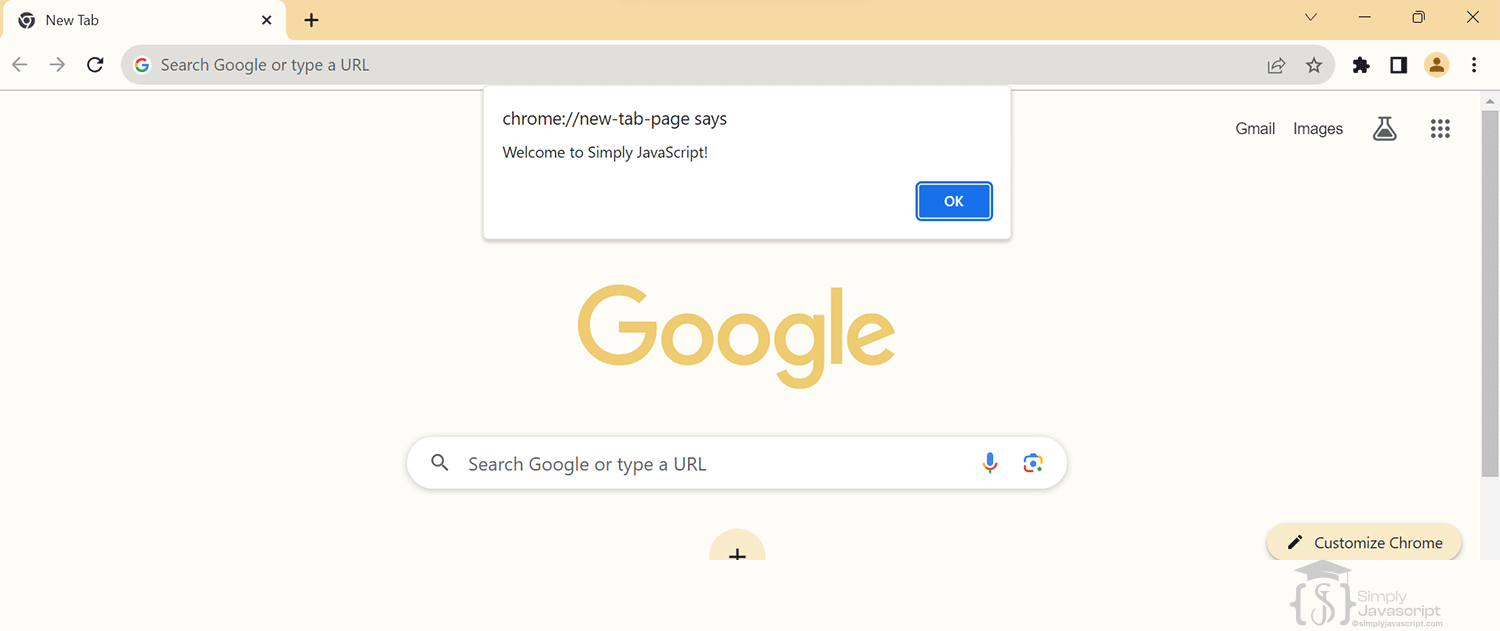
The confirm box in JavaScript, invoked using window.confirm(), displays a dialog box with an OK and Cancel button, prompting the user for a confirmation response. In JavaScript confirm() method—it's like asking your user a yes-or-no question with an "OK" and a "Cancel" button. When you use confirm(), you're creating a confirmation dialog box. For example:
let result = confirm("Do you want to continue?");
This pops up a little window asking your user if they want to proceed. They can then click "OK" or "Cancel." confirm() returns true if the user clicks "OK" and false if they click "Cancel" or simply close the dialog.
if (result) {
console.log("User clicked OK!");
} else {
console.log("User clicked Cancel or closed the dialog!");
}
If result is true, it means the user clicked "OK," and if it's false, they either clicked "Cancel" or closed the dialog box without clicking anything. It’s like getting a response from your user—pretty handy for making decisions in your code based on what they choose!
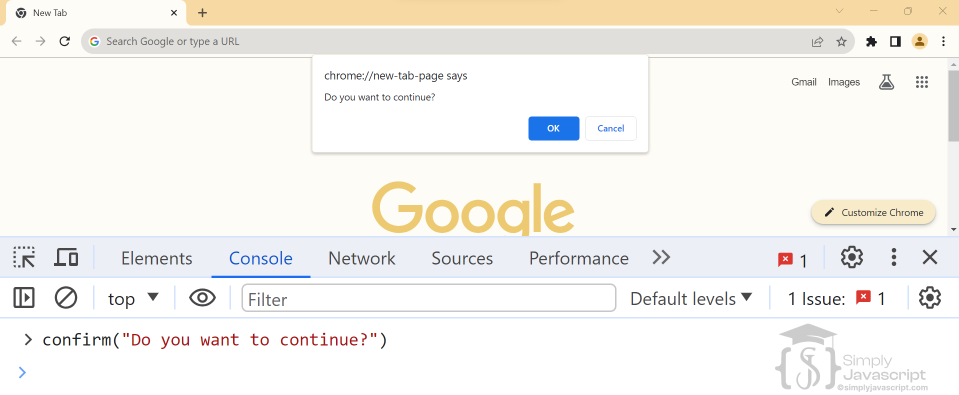
The prompt box in JavaScript, initiated using window.prompt(), prompts the user to enter input within a dialog box, allowing data entry or user interaction. Here’s how it works:
let answer = prompt("What is your name?");
console.log(answer);
When this code runs, a prompt box appears, asking the user for their name. They can then type something in and hit "OK" or simply click "Cancel." Now, prompt() does two things, if the user clicks "OK" after typing in something, the text they entered is stored in the variable answer. But if they click "Cancel" or close the dialog, it returns null.
So, that console.log(answer) will show the text the user typed in if they clicked "OK," or it’ll show null if they clicked "Cancel" or closed the prompt without typing anything. It’s like having a little chat window where you ask a question, and depending on how the other person responds (or if they don’t respond at all), you get a different outcome. Great for getting user input and making your code interactive!
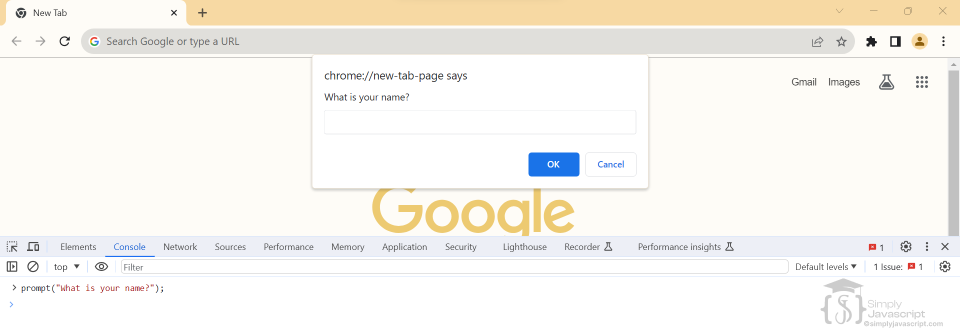
Note: When you use the prompt() box in JavaScript, it returns the user's input as a string. So, if you need it as a different type, like a number, you'll have to typecast it to specific type.
alert():
- Displays a pop-up with a specified message and an 'OK' button.
- Ideal for quick warnings or alerts to users.
- Use it wisely to avoid being overly intrusive.
confirm():
- Prompts a yes-or-no question with 'OK' and 'Cancel' buttons.
- Returns true if 'OK' is clicked and false if 'Cancel' or the dialog is closed.
- Useful for decision-making based on user input.
prompt():
- Asks the user for input through a pop-up box.
- Stores the entered text if 'OK' is pressed after typing; returns null if 'Cancel' or the prompt is closed.
- Great for interactive user input and handling different responses.
- Explain alert.
- What are the limitations of using an alert box in terms of user interaction and customization?
- Explain the functionality of a confirm box in JavaScript. How does it differ from an alert box, and when might you use it in a web application?
- Explain the usage of a prompt box in JavaScript. How does it allow users to input data, and what are some considerations when using it in your code?
- Describe scenarios where using a prompt box would be appropriate for user interaction within a web application.
- Compare and contrast the alert, confirm, and prompt boxes in JavaScript in terms of their functionality, user interaction, and when to use each type.