Control Flow
Conditional statements in programming execute different actions based on whether a specified condition evaluates to true or false, altering program flow accordingly. Think of them as pathways that direct the flow of your program or like traffic signals for your code—they guide its flow based on conditions you set. Let's break down what they do using examples. Take this snippet:
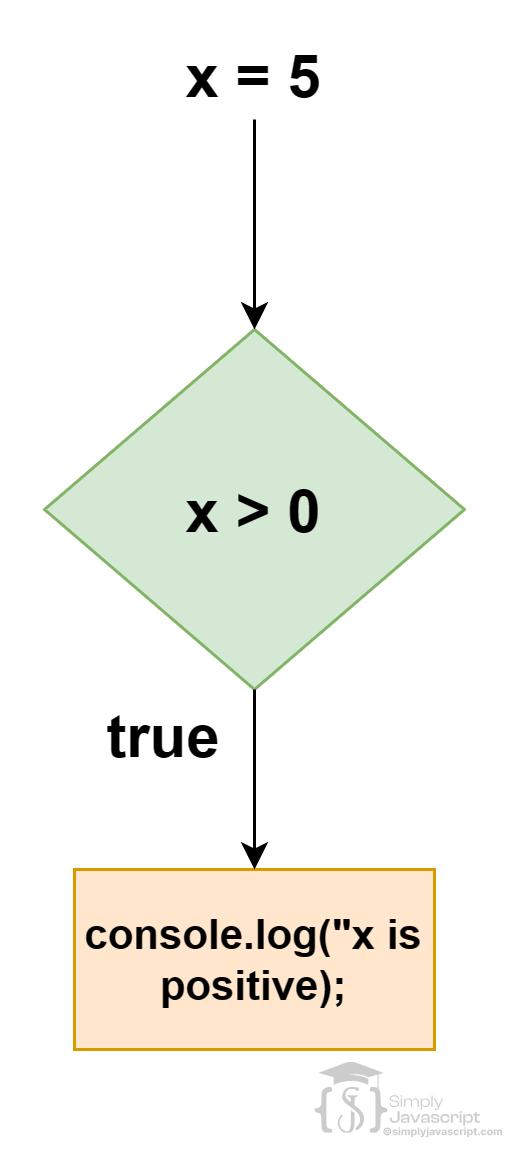
let x = 5;
if (x > 0) {
console.log("x is positive");
}
Here, the if statement checks if x is greater than 0. If true, the code inside the curly braces runs. If x isn't greater than 0, the code is skipped. You can also pair it with an else statement for an alternative action:
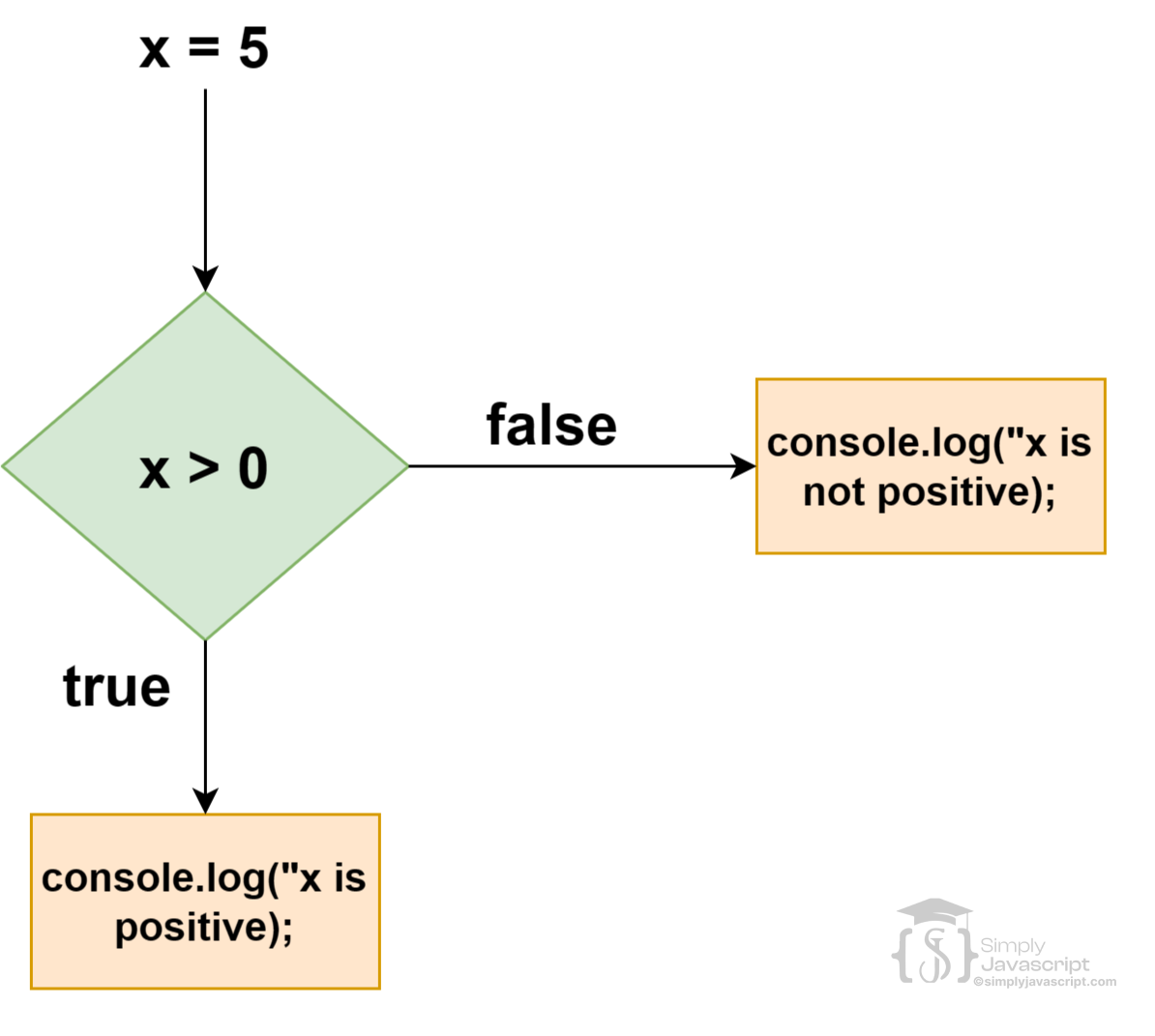
let x = 5;
if (x > 0) {
console.log("x is positive");
} else {
console.log("x is not positive");
}
In this setup, if x is greater than 0, the first message logs. If not, the second message does. Now, things get more interesting with else if. It's like having a bunch of options:
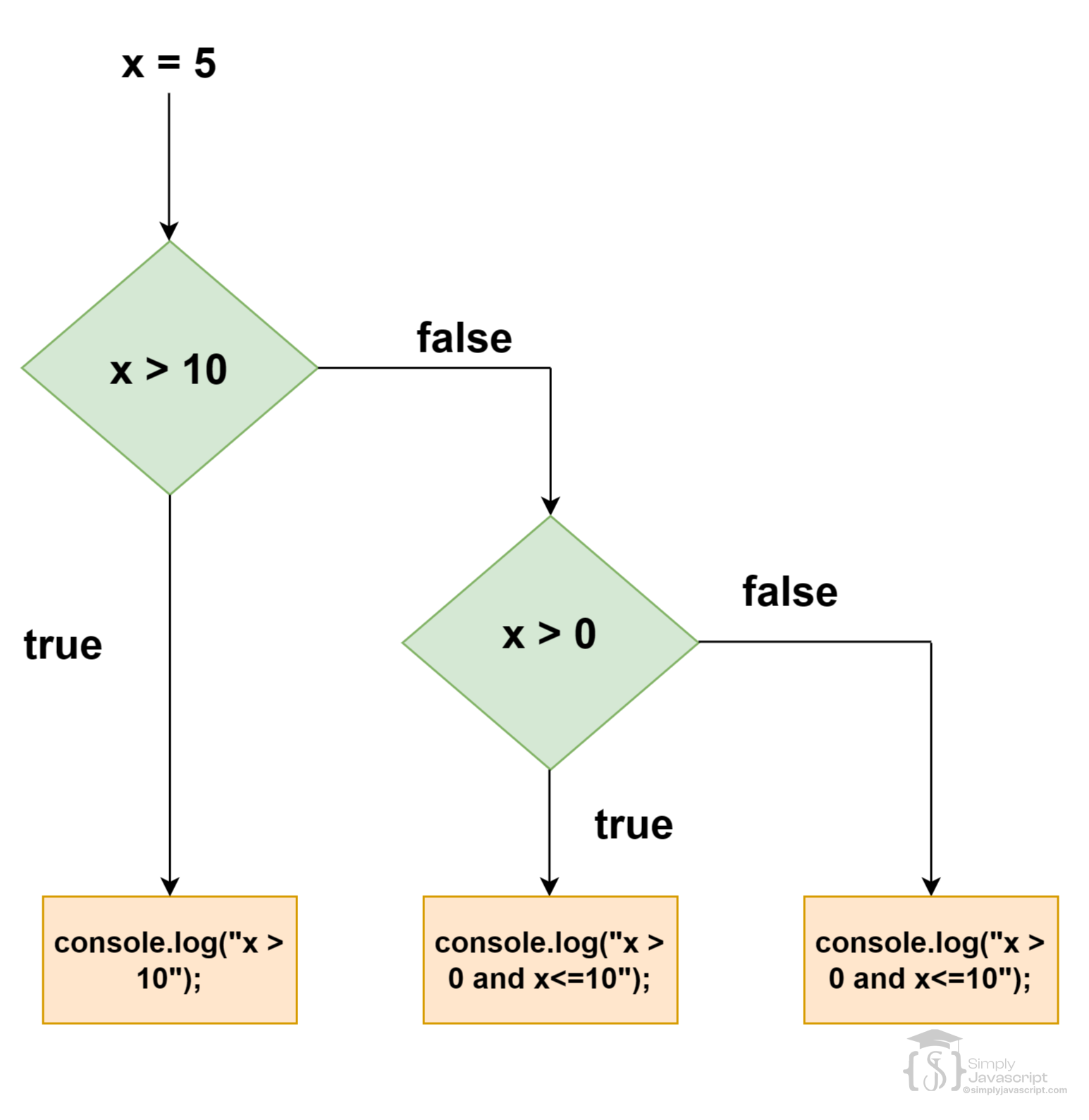
let x = 5;
if (x > 10) {
console.log("x is greater than 10");
} else if (x > 0) {
console.log("x is greater than 0 but less than or equal to 10");
} else {
console.log("x is less than or equal to 0");
}
Else and else if statements in JavaScript must always follow an if statement. They're dependent on an initial if condition to work properly. Without an initial if, standalone else or else if statements won't function as expected in JavaScript.
//For instance, this structure won't work because the else if is not preceded by an if statement:
else if (condition) {
// code block
}
//Similarly, standalone else statements without an if won't function either:
else {
// code block
}
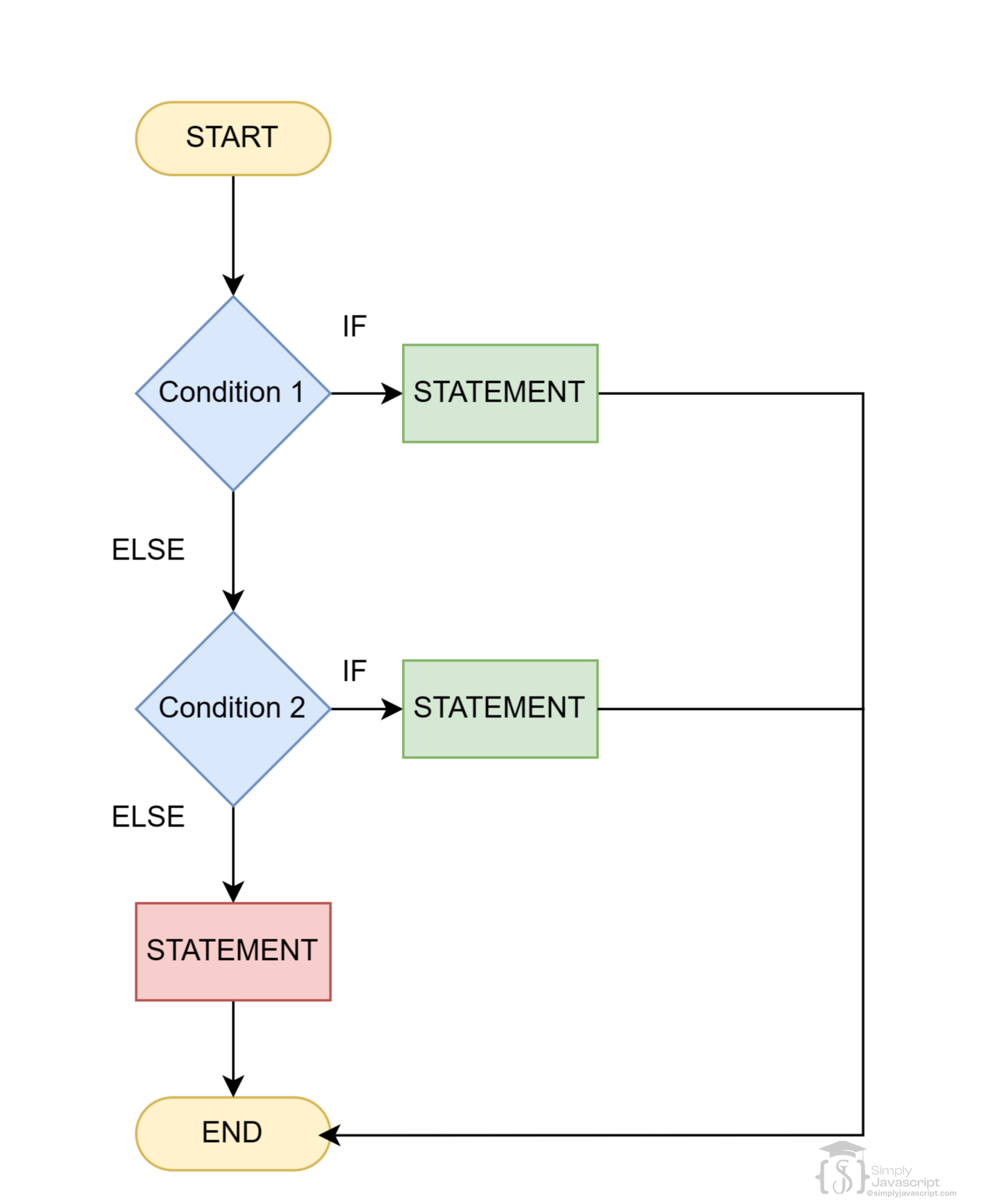
In JavaScript, the logical operators && and || can be used to group multiple conditions together and evaluate them as a single Boolean expression. The && operator (logical AND) returns true only if both conditions are true. If either condition is false, the expression will evaluate to false. For example:
let x = 5;
if (x > 0 && x < 10) {
console.log('x is between 0 and 10');
}
The || operator (logical OR) returns true if at least one of the conditions is true. If both conditions are false, the expression will evaluate to false. For example:
let x = 30;
if (x < 0 || x > 10) {
console.log('x is not between 0 and 10');
}
It's worth noting that the logical operators && and || are short-circuit operators, which means that the second operand is only evaluated if the first one does not determine the outcome of the expression. For example, in the case of x > 0 && x < 10, if x is greater than 0, the second operand will be evaluated, otherwise the expression returns false without evaluating the second operand.
Nested if-else statements are the sequence of conditions within conditions, each one encapsulated within the other. Nested if-else statements help break down complex conditions into more manageable steps. if you have conditions that need further conditions to be checked. That's where nesting comes into play. Here's an example:
if (x > 0) {
if (x < 10) {
console.log('x is between 0 and 10');
} else {
console.log('x is greater than or equal to 10');
}
} else {
console.log('x is less than or equal to 0');
}
Here, we first check if x is greater than 0. If it is, we dive into another check—whether it's less than 10. If both these conditions pass, the message 'x is between 0 and 10' gets logged. But, if x isn't less than 10 in the nested if, we catch it with the else statement inside. And if x is not greater than 0 initially, we directly go to the else block of the first if.
- What is control structure in programming?
- What is conditional statement in programming?
- Explain the purpose and syntax of the if statement in JavaScript. How is it used to execute code conditionally based on a specified condition?
- Describe the role of the else statement in JavaScript. When is it used, and what happens when the condition in the if statement evaluates to false?
- Explain the else if statement in JavaScript. How does it differ from if and else statements, and when might you use it in your code?
- Explain how you can group multiple conditions using logical operators (AND, OR) within an if statement in JavaScript. Provide examples of using logical operators to combine conditions.
- Describe the concept of nested if-else statements in JavaScript. When is nesting used, and can you provide an example demonstrating nested conditional logic?
- Compare and contrast the if, else if, else statements, and nested if-else structures in terms of their usage and scenarios where each is most appropriate.